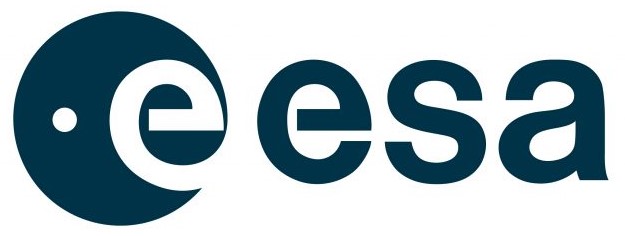
European Space Agency¶
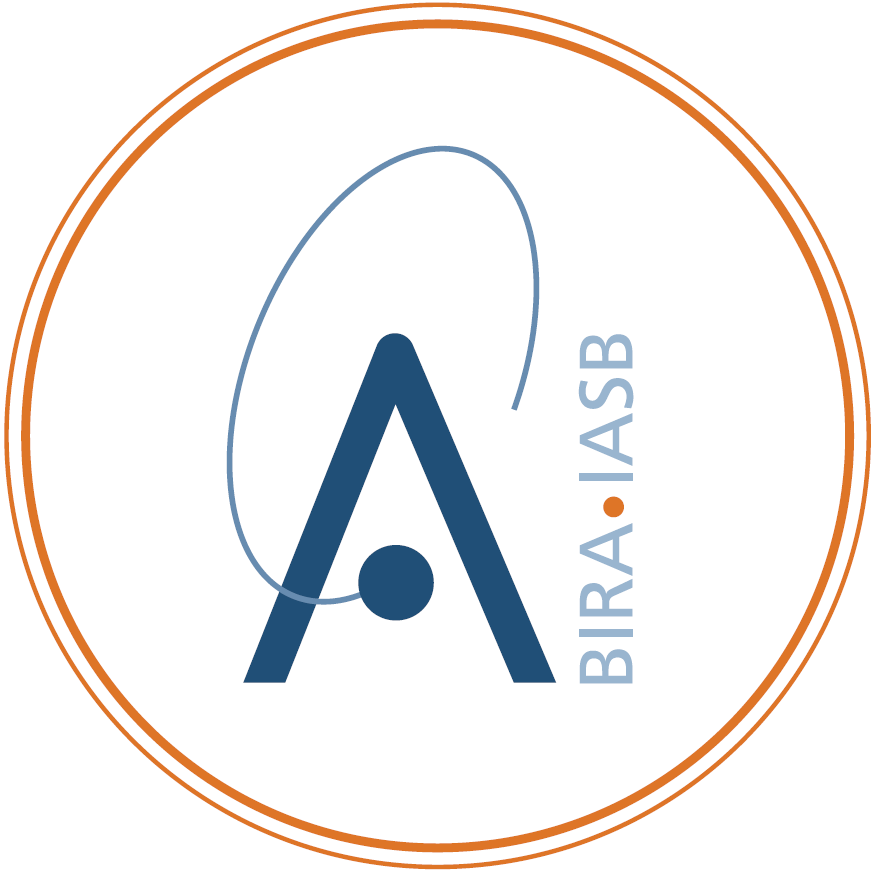
Royal Belgian Institute for Space Aeronomy¶

G.06 - How to start using the UNILIB library ?¶
Answer¶
The functionality of the library has to be accessed with the help of a FORTRAN programme or a IDL routine. A sample of FORTRAN programme is given in the illustration, as well as the corresponding IDL programme. Note that the Subroutines contains help for each subroutine, including synopsis, description, dependencies and list of reported bugs.
Initialization¶
Before most of the library subroutines can be used, the different common blocks of the library have to be initialized. The following initalisation subroutines are provided inside the library:
Main subroutines¶
The subroutines of the library can be divided into three sets: (1) the main subroutines, (2) the internal subroutines and (3) the miscellaneous subroutines. The internal subroutines are subroutines called by other subroutines of the library and should not be called by the application. The main subroutines are top-level subroutines, they include:
ua630()
, to evaluate the atmospheric number and mass densities;
ua636()
, to evaluate the atmospheric number or mass densities weighted by cross sections;
ul220()
, to evaluate \(B_m\), L, K for a set of field line segments passing through a given position;
ud310()
, to trace a magnetic drift shell;
ud330()
, to evaluate the third invariant;
uf420()
, to trace a magnetic field line segment passing through a given position;
ut980()
, to print the library error messages.The miscellaneous subroutines are subroutines called by other subroutines of the library but that users can also use directly, e.g.
um523()
,um524()
orut540()
. The help pages for the main subroutines are a good starting point to learn about the library. Another starting point in the understanding of the library are the examples provided in the documentation.
Component identifiers¶
In the library, each component has a unique identifier. The FORTRAN common block components of the library are identified by the two characters
UC
followed by three digits. The FORTRAN subroutine components of the library are identified by two characters, related to the functionality of the component, followed by three digits. The charactersUL
,UF
,UD
,UM
,UA
, andUT
correspond to geomagnetic labels, field line tracing, drift shell tracing, magnetic models, atmospheric models, and general tools, respectively. Each component of the library is uniquely defined by its 3-digit code. For the variables used in the library, the first character of the identifier indicates the type of the variable. The correspondences between the first character of the identifier and the FORTRAN type of the field are:
A-H, P-Y for REAL*8;
I, J, K, N for INTEGER*4;
L for CHARACTER*(*);
M for (FORTRAN 90) derived types;
Z for EXTERNAL.
Error diagnostics¶
When an error occurs in a subroutine of the library, the error diagnostic is returned by the way of a negative integer value of the form
-dddii
. The three first digits (ddd
) are set to the 3-digits of the subroutine where the error occurs. The two last digits (ii
) are used to differentiate errors inside a same subroutine. The errors are documented in the Diagnostics section of each subroutine. Note that some subroutines get an error flag as argument only to pass the error flag of their dependents.
Illustration¶
In the FORTRAN programme below, a magnetic field line segment is computed and printed. The field line segment is passing through the GEO location [2,000 km, 40 deg N, 30 deg E] and limited by a magnetic field intensity of 0.4 Gauss. The library is initialized with the IGRF-95 geomagnetic field model.
PROGRAM sample
C
INCLUDE 'unilib.h'
INTEGER*4 kunit, kinit, ifail, kint, kext, nfbm
CHARACTER*32 lbint, lbext
REAL*8 year, param(10), amjd, fbm(10), falt
TYPE(zgeo) mpos
C
DATA kunit, kinit, kint, year/ 6, 1, 0, 1995.0d0/
DATA kext, amjd, param/ 0, 0.d0, 10*0.0d0/
DATA nfbm, falt, fbm/ 1, -999., 0.4, 9*0./
C
C Initialization
C
CALL UT990 (kunit, kinit, ifail)
IF( ifail .LT. 0 )STOP
CALL UM510 (kint, year, lbint, kunit, ifail)
IF( ifail .LT. 0 )STOP
CALL UM520 (kext, amjd, param, lbext, kunit, ifail)
IF( ifail .LT. 0 )STOP
C
C Body part
C
mpos.radius = 8371.2
mpos.colat = 50.
mpos.elong = 30.
CALL UF420 (mpos, fbm, nfbm, falt, ifail)
IF( ifail .LT. 0 )STOP
C
C Result Printing
C
CALL UT991 (kunit, ifail)
IF( ifail .LT. 0 )STOP
C
END
The same program but written with the IDL command language is presented below.
; sample.pro
solib = getenv( 'UNILIB')
IF strlen(solib) eq 0 THEN MESSAGE, 'The UNILIB environment variable has to be '+ $
'defined before running idl'
MESSAGE, 'File '+ strtrim( solib, 2)+ ' linked', /informational
;
; Data
;
kunit= 6L & kinit= 1L & kint= 0L & year= 1995.0D0
kext= 0L & amjd= 0.0d0 & param= DBLARR(10)
nfbm= 1L & falt= -999.0d0
fbm= DBLARR(nfbm) & fbm(*)= [ 0.4d0 ]
;
; Initialization
;
version = 0L
status = CALL_EXTERNAL( solib, 'idl_ut990_', -6L, 1L, version)
IF version LT 0 THEN MESSAGE, 'Unable to initialize'+ $
' the Unirad Library'
MESSAGE, 'Unirad Library v'+ STRCOMPRESS( STRING( $
version*0.01, format= '(f10.2)'), /remove_all), $
/informational
lbint = '12345678901234567890123456789012'
ifail = 0L
status = CALL_EXTERNAL( solib, 'idl_um510_', kint, year, $
lbint, kunit, ifail)
IF ifail LT 0 THEN MESSAGE, 'Error'+ STRING(ifail)+' in UM510'
lbext = '12345678901234567890123456789012'
ifail = 0L
status = CALL_EXTERNAL( solib, 'idl_um520_', kext, amjd , $
param, lbext, kunit, ifail)
IF ifail LT 0 THEN MESSAGE, 'Error'+ STRING(ifail)+' in UM520'
;
; Body part
;
mpos = {zgeo, radius: 8371.2d0, $
colat: 50.0d0, $
elong: 30.0d0}
ifail = 0L
status = CALL_EXTERNAL( solib, 'idl_uf420_', mpos, fbm, nfbm, $
falt, ifail)
IF ifail LT 0 THEN MESSAGE, 'Error'+ STRING(ifail)+' in UF420'
;
; Result Printing
;
ifail = 0L
status = CALL_EXTERNAL( solib, 'idl_ut991_', kunit, ifail)
IF ifail LT 0 THEN MESSAGE, 'Error'+ STRING(ifail)+' in UT991'
;
END
See also¶
UNILIB/trunk/